The Fakoli List View classes provide display handling of a set of records in a table list format or grouped into an expandable tree control. The List View classes are designed to work with data model objects derived from the DataItem or InnerJoinResult base classes, making them ideal for listing search results or generated reports. They also provide the capability to download the tabular data in Excel format with a minimum of additional coding.
Data List View
The Data List View displays a set of records in table format with sortable and filterable columns that can be set to display a set number or records per page. The following script creates a data list view of the task table:
$tasks = query(Task, "ORDER BY title");
$table = new DataListView($tasks, "tasks", "list", true, 10, true);
$table->column("Task Title", "{title}", true);
$table->column("Project", "{Project.project_name}", true);
$table->column("Due Date", "{due_date}", true);
$table->cssStyle = "width: 70%;";
require_once "head.inc"; // header, styles, javascript, mootools
echo $table->writeScript();
$table->drawView();
- $tasks = query(Task, "ORDER BY title");
- $table = new DataListView($tasks, "tasks", "list", true, 10, true);
- $table->column("Task Title", "<a href="/task_form.php?task_id={task_id}">{title}</a>", true);
- $table->column("Project", "{Project.project_name}", true);
- $table->column("Due Date", "{due_date}", true);
- $table->cssStyle = "width: 70%;";
- require_once "head.inc"; // header, styles, javascript, mootools
- echo $table->writeScript();
- $table->drawView();
$tasks = query(Task, "ORDER BY title");
$table = new DataListView($tasks, "tasks", "list", true, 10, true);
$table->column("Task Title", "<a href="/task_form.php?task_id={task_id}">{title}</a>", true);
$table->column("Project", "{Project.project_name}", true);
$table->column("Due Date", "{due_date}", true);
$table->cssStyle = "width: 70%;";
require_once "head.inc"; // header, styles, javascript, mootools
echo $table->writeScript();
$table->drawView();
The code above retrieves all the task records, creates a DataListView of those records, and turns on sorting and filtering. The script creates a table with 3 columns: title, project name, and due date. The script creates the table shown below:
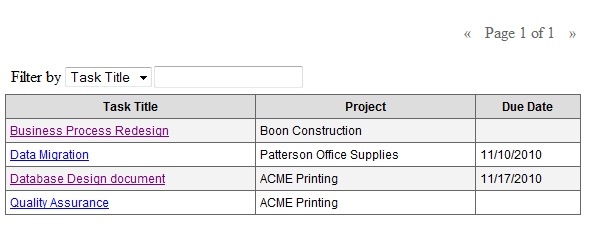
The data is initially sorted by the task title since that was the order of the query. If the user clicks on the Project column heading, the data will be sorted by project name, as follows:
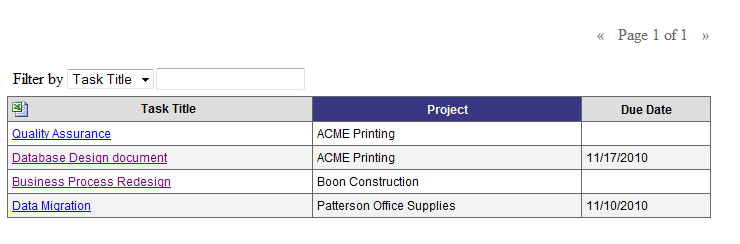
The data for column values can be derived from: any of the fields in the set of retrieved records; any field that can be retrieved through a relation or function defined in the data model; or through a callback function. The following table describes the notation for each retrieval type. Note that the first three are passed to the column function as a string whereas the callback is not.
Type | Notation | Example |
Field within the retrieved record |
"{field}" |
{title} |
Field in a related table, defined in the data model |
"{Relation.field}" |
{Project.project_name} |
Function defined in the data model |
"{functionName()}" |
{GetProjectName()} |
callback function |
functionName |
getStatus where getStatus is a function defined in the calling script or one of its include files. |
You can find more information on the object formatting syntax in Chapter 3.
Grouped Data View
The Grouped Data View displays sets of records grouped by a specified field. If we wanted to display tasks grouped by their project, we would create a Grouped Data View as follows:

The following view shows the Grouped Data View with one node expanded:
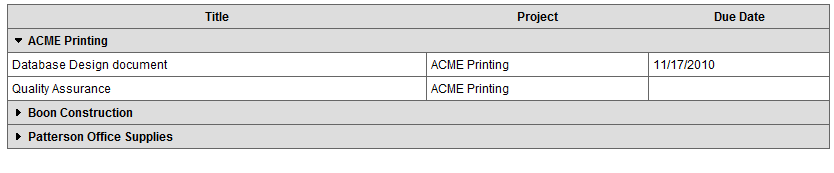
Creating a Grouped Data View is extremely simple, and makes use of the GroupedQuery feature discussed in Chapter 2. Construction of the groups can be accomplished either manually (by creating each group in turn) or by making use of the GroupedDataListView::groupBy() method, which can be used to automatically populate the groups based on a list of DataItems. An example of this second method is given below:
$tasksByProject = GroupedQuery::create(Task, "ORDER BY title", "site_id")->execute();
$projects = Query::create(Project, "ORDER BY project_name")->execute();
$table= new GroupedDataListView($tasksByProject , "tasks");
$table->column("Task Title", "{title}", true);
$table->column("Project", "{Project.project_name}", true);
$table->column("Due Date", "{due_date}", true);
$table->mode = "tree";
$table->groupBy($projects);
$table->cssStyle = "width: 100%";
$script = $table->writeScript();
$table->drawView();
- $tasksByProject = GroupedQuery::create(Task, "ORDER BY title", "site_id")->execute();
- $projects = Query::create(Project, "ORDER BY project_name")->execute();
- $table= new GroupedDataListView($tasksByProject , "tasks");
- $table->column("Task Title", "<a href="/task_form.php?task_id={task_id}">{title}</a>", true);
- $table->column("Project", "{Project.project_name}", true);
- $table->column("Due Date", "{due_date}", true);
- $table->mode = "tree";
- $table->groupBy($projects);
- $table->cssStyle = "width: 100%";
- $script = $table->writeScript();
- $table->drawView();
$tasksByProject = GroupedQuery::create(Task, "ORDER BY title", "site_id")->execute();
$projects = Query::create(Project, "ORDER BY project_name")->execute();
$table= new GroupedDataListView($tasksByProject , "tasks");
$table->column("Task Title", "<a href="/task_form.php?task_id={task_id}">{title}</a>", true);
$table->column("Project", "{Project.project_name}", true);
$table->column("Due Date", "{due_date}", true);
$table->mode = "tree";
$table->groupBy($projects);
$table->cssStyle = "width: 100%";
$script = $table->writeScript();
$table->drawView();
The GroupedDataListView can operate in three modes:
- tree - in this mode, each group can be opened and closed independently of the others.
- accordion - only one group can be open at a time. Clicking to open a second group closes the first.
- fixed - all groups are shown as open, and cannot be closed.
Exporting Data Views to Excel
The tables produced by both the DataListView and GroupedDataListView classes can be exported to an Excel spreadsheet. To do this, the programmer only has to specify an output name for the Excel file, as follows:
$table->excelFile = "tasks.xls";
- $table->excelFile = "tasks.xls";
$table->excelFile = "tasks.xls";
An Excel icon is then displayed in the top left of the table:
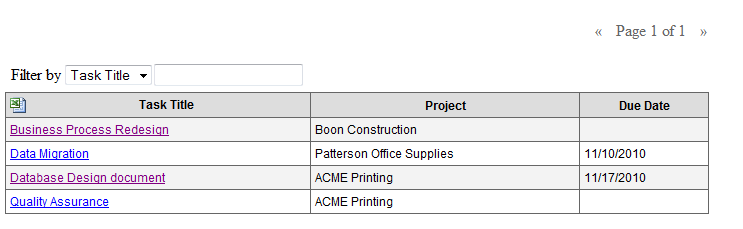
When the Excel icon is clicked, the user can choose to open or save the data in spreadsheet format to a specified file name.
Chapter 9. User Interface Components »
« Chapter 7. Search Forms